SOCIAL
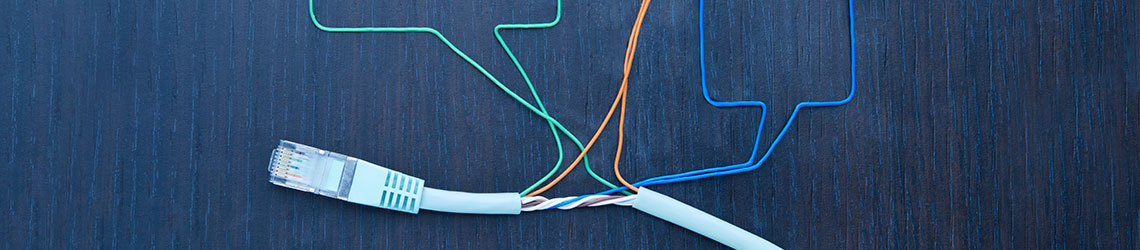
Connecting to Facebook
<script>
$.getJSON(
'http://bytomasz.com/social/facebook/250253191483',
function(data){
console.log(data);
}
);
</script>
Additional notes
To get your facebook ID just go to this page and submit your username to get it. I'm also using jquery.dateFormat.js and moment.js to get relative time date in javascript from either feed. Finally, I'm using dotdotdot.js for ellipsis functionality.
Putting it together
Full Code
<script> if($('#twitter').length){ var twitter = 'ToloPoland'; $.getJSON( 'http://bytomasz.com/social/twitter/'+twitter, function(data){ var tweet = data.statuses[0]; var media = tweet.entities.media[0]; var image = media.media_url; var string = $.format.date(tweet.created_at, "yyyy-MM-dd HH:mm Z") $('#twitter time').text(moment(string,"YYYY-MM-DD HH:mm Z").fromNow()); $('#twitter p').text(tweet.text); $('#twitter p').prepend('<img src="'+image+'">'); $('#twitter a.s-link').attr('href', "https://twitter.com/@"+twitter); $("#twitter p").dotdotdot({ watch:true }); } ); $('html').on('click', '#twitter', function(e){ e.stopPropagation(); var url = $('#twitter a.s-link').attr('href'); var win = window.open(url, '_blank'); win.focus(); }); } if($('#facebook').length){ var facebook = '176509522402116'; $.getJSON( 'http://bytomasz.com/social/facebook/'+facebook, function(data){ var post = data.responseData.feed.entries[0]; var date = new Date(post.publishedDate); var m = date.getMonth() > 9 ? String(date.getMonth() + 1) : 0 + String(date.getMonth() + 1); var d = date.getDate() > 9 ? String(date.getDate()) : 0 + String(date.getDate()); var hr = date.getHours() > 9 ? String(date.getHours()) : 0 + String(date.getHours()); var mi = date.getMinutes() > 9 ? String(date.getMinutes()) : 0 + String(date.getMinutes()); var zn = date.getTimezoneOffset(); var string = String(date.getFullYear())+'-'+m+'-'+d+' '+hr+':'+mi+' '+zn; var image = post.thumb; $('#facebook time').text(moment(string,"YYYY-MM-DD HH:mm Z").fromNow()); $('#facebook p').text(post.content); $('#facebook p').prepend('<img src="'+image+'">'); $('#facebook a.s-link').attr('href', "https://facebook.com/"+facebook); $("#facebook p").dotdotdot({ watch:true }); } ); $('html').on('click', '#facebook', function(e){ e.stopPropagation(); var url = $('#facebook a.s-link').attr('href'); var win = window.open(url, '_blank'); win.focus(); }); } </script>
<style> #twitter { background:#5EA9DD; } #facebook { background:#3B5998; } #twitter, #facebook { position:relative; padding:20px; cursor:pointer; } #twitter p, #facebook p { font-size:22px; line-height:1.3em; color:#fff; height:84px; overflow:hidden; margin:0; padding:0; } #twitter time, #facebook time { display: block; margin-top: 15px; color: rgba(255,255,255,0.7); text-align:left; } #twitter i.fa, #facebook i.fa { position: absolute; right:20px; bottom:12px; font-size:50px; color: rgba(255,255,255,0.3); } #twitter h5, #facebook h5 { font-size: 12px; color:#fff; text-align:left; margin-top:20px; } #twitter img, #facebook img { float: left; height: 84px; margin-right: 12px; border:2px solid #fff; } #twitter .s-link, #facebook .s-link { display:none; } </css>
<div id="twitter"> <p></p> <time></time> <i class="fa fa-twitter"></i> <h5>New Tweets</h5> <a href="" class="s-link" target="_blank">LINK</a> </div> <div id="facebook"> <p></p> <time></time> <i class="fa fa-facebook"></i> <h5>Facebook Feed</h5> <a href="" class="s-link" target="_blank">LINK</a> </div>